How to Code Like a Pro
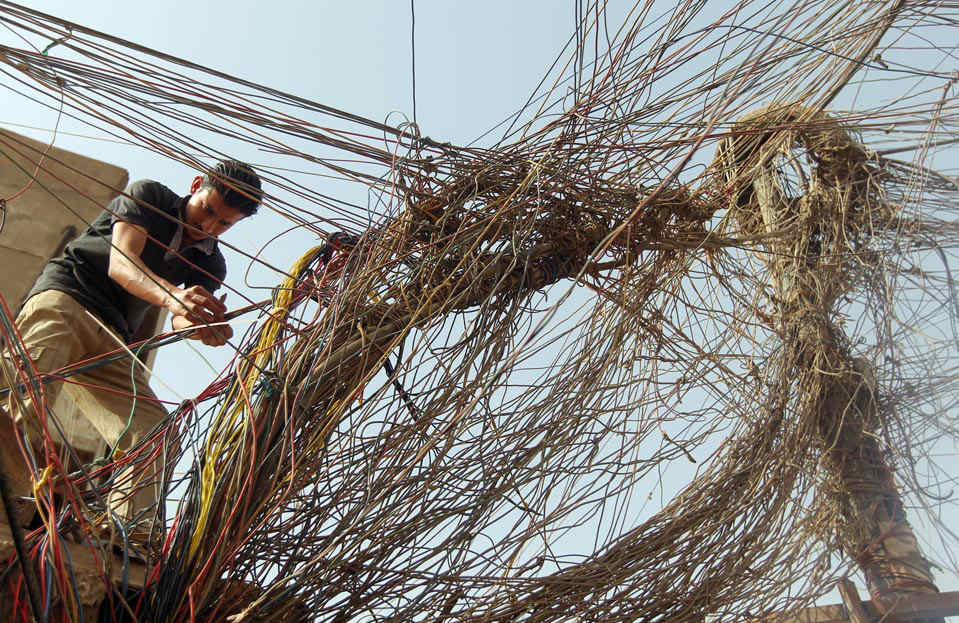
So you are a programmer, huh? You want to write cool and really sophisticated code. Like a pro? Here is my list that can help you to get started.
Choose programming language like a pro
First, you need to choose a language that you will use to implement your apps. Your language of choice defines how good of a programmer you are.
- Use new and exotic programming languages. Real pros use cool languages, not that mainstream crap that everyone else is using. Try Haxe or EmberScript. And don’t forget to convince everyone that it is way better than anything they have used before.
- Use old, time-tested programming languages. All those new hippie languages are not for everyone. If you are old-school - use something proven by time. Like C++98, Perl or PHP3.
- Stick to your language of choice and never betray it. Use it for everything and refuse to learn any other heresy.
Design application like a pro
Design is the most important part of your application. Your design must be complex and sophisticated. Only real pros should be able to understand it.
- Use all design patterns that you know. You just finished the Gang of Four book, time to put it into practice, right? Use as many patterns as you can remember.
- Learn even more patterns and use them. The more patterns you use in your application, the more of a pro you are.
- Use Singleton as much as possible. This is your most important pattern. There can be only one AbstractAdapterBuilderFactory in your application, right?
- Create your own format for configuration files. It will be 100 times better than existing ones. If you feel lazy, just use XML.
- Download configurations from remote server. And make sure part of it is generated.
- Use Frameworks for everything. Logging framework, object instantiation framework, annotation framework, frameworks of frameworks.
- Especially, dependency injection frameworks. Even if your application consists of 3 classes.
- Design your application to have as many layers as you can. The more, the better.
- Add more dependencies. If you want your application to be dependable, your components must depend on each other. All of them. Also, depend on as many libraries as you can. And don’t forget to add a circular dependency or two.
Implement like a pro
Design of application is important, but your implementation is the first thing others will see. Make sure they realize that they see pro-level implementation. Here is how you can achieve it.
- Create interfaces for every single class. Interfaces are cool. Every class should have an interface and only one default Impl class.
- Make all your classes generic. Specialization is for noobs only. What if your rectangle needs to have 5 corners?
- Use names that only you can understand. ‘avr_gdf_qww’ is a totally legit name for a variable. ‘do_stuff()' is a good name for a function.
- Never alow logic of your function to be obvious from the first glance. Use code branching, nested ‘if’ conditionals, and switches as much as possible.
- Use side effects. If your function adds two variables, why don’t it also increment the first one? It should also sort global array and flip a few global boolean flags.
- Modify input arguments in your function. Your functions must show authority, or no one will really respect them. They can do whatever they want with variables they are given.
- Make everything implicit. The more is done by ‘magic’ - the less others will understand and the smarter you will look to them.
- Generate as much code as you can. Better, generate code that will generate code.
- Use try/catch everywhere. Wrap any function call that you doubt in try/catch. Better wrap all your logic in try/catch and don’t let any single exception to slip away.
- Use exceptions to control execution flow. They are almost as good as GOTO statement.
- Create custom exceptions for everything. Built-in exceptions are just not good enough.
- Put all your code into one file, or into one giant class if your programming language allows that. Your code must be monstrous and instill fear.
- Use long functions. Size matters! The longer the function, the more sophisticated it is, the better programmer you are. No less than 800 lines.
- Re-use variables for performance optimization. If you already have a variable called ‘square_root’, why can’t it contain local time a few lines down? It is already there, right?
- Global variables are your friends. Especially mutable ones.
- Implement your own copy, equals, to string functions. You can do it better than the standard library. I believe in you!
- Pass as many arguments to functions as you can. If constructor has less than 12 arguments, no one will take it seriously.
- Any good function must take at least 3 boolean parameters. And a callback function.
- Use boolean flags for everything.
- Use inheritance intensively. All your classes should derive from a common base class.
- Use multithreading for everything. Write your own syncroniztion primitives.
- Write your own serializers for everything and develop your own serialization formats. JSON is so 2000.
- Handle dates and time conversion yourself. Never trust standard implementations. Who knows what they put in there?
- Make all your fields and member variables public. You have no secrets.
- Don’t ever write unit tests. Those are for QA. Good programmers write good code right away.
- Never refactor. Masterpiece of your creation should live forever for future generations to behold.
Summary
If you follow these simple commandments, my friend, you will be the most respected coder in your company. Only you will be able to understand your code, let alone change it. Bonus points if after a week even you cannot understand it!
What are your secrets to code like a pro? Share in comments below!
P.S. It would have been funny if it wasn’t sad. Unfortunately, I had a ‘pleasure’ to encounter all those and many more throughout my career.
Title image is from New York Times